What are the different ways to create objects in JavaScript?
Last Updated on :February 26, 2024
In javascript there are five different ways to create an object
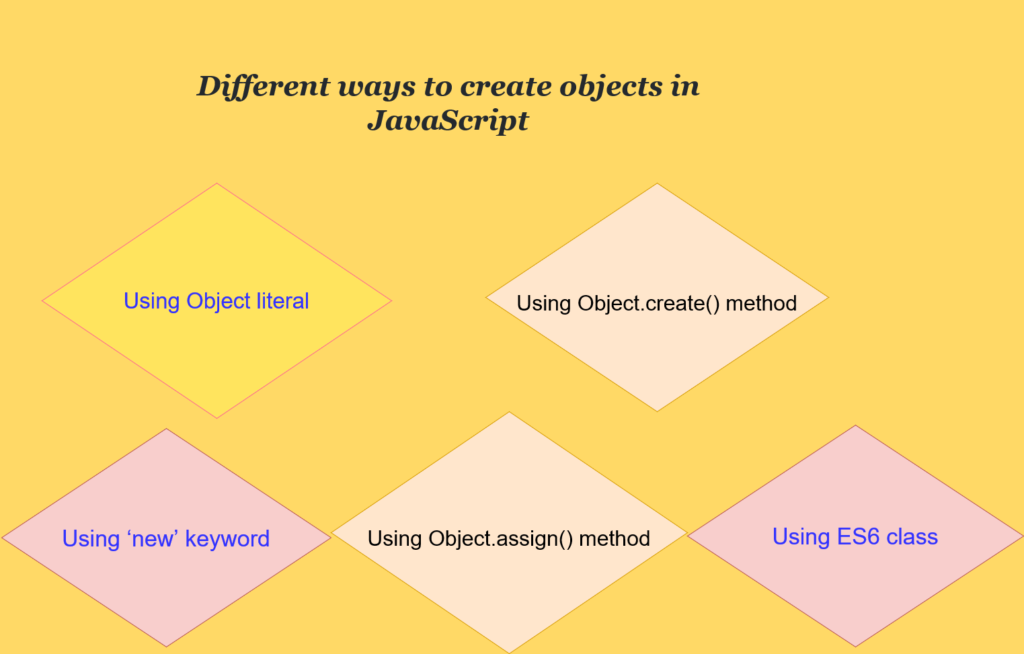
- Using Object literal
- Using the ‘new’ keyword
- Using Object.create method
- Using Object.assign()
- Using ES6 Classes
1. Object literal
An object literal is a set of key-value pair separated by ‘:’ inside a set of curly braces ({ }) . This is the simplest and most popular way to create objects in JavaScript.
You can create an object literal as shown in below
var empObj= {
firstName: 'Rakesh',
lastName: 'Panigrahi'
};
console.log(JSON.stringify(empObj));
Once you created the object , you can add properties dynamically in an object. Here we add the dynamic property empObj.email and empObj.salary
var empObj= {
firstName: 'Rakesh',
lastName: 'Panigrahi'
};
empObj.email = ‘[email protected]’; //dynamic property
empObj.salary = 2000; //dynamic property
console.log(JSON.stringify(empObj));
2. Using the ‘new’ keyword
The new keyword is used in javascript to create an object from a constructor function.
You can create an object using new keyword as shown in below example
function Employee(firstName, lastName, email) {
this.firstName = firstName;
this.lastName = lastName;
this.email = email;
}
var empObj = new Employee('Rakesh','Panigrahi','[email protected]');
console.log(JSON.stringify(empObj));
3. Object.create() method:
The Object.create() method creates a new object by passing the prototype object as a parameter.
Object.create() takes 2 arguments and returns a new object.
- The first argument is a mandatory object that serves as the prototype of the new object to be created.
- The second argument is an object which will be the properties of the newly created object
Example
var companyObj = { company: 'XYZ Company' };
var empObj = Object.create(companyObj, {
name:
{ value: 'Rakesh P' }
})
console.log('Company Obj:', JSON.stringify(companyObj));
console.log('Employee Name:', empObj.name);
In above example the newly created empObj will inherit all prototype of companyObj
4. Object.assign() method
The Object.assign() copies all enumerable and own properties from the source objects to the target object.
If we want to create an object that needs to have properties from more than one object then we can achieve this by using Object.assign() method.
In the below example we have merged person name object and person details object.
var personName = {
firstName: 'Rakesh',
lastName: 'Panigrahi'
};
var personDetails = {
height : '180 cm',
weight : '78 kg',
age : '30 years'
};
const personObj = Object.assign({}, personName, personDetails);
console.log(JSON.stringify(personObj));
{
"firstName": "Rakesh",
"lastName": "Panigrahi",
"height": "180 cm",
"weight": "78 kg",
"age": "30 years"
}
5. Using ES6 classes
ES6 introduces class features to create the new object . Let’s look into the code below.
class Person {
constructor(firstName,lastName) {
this.firstName = firstName;
this.lastName = lastName;
}
}
var object = new Person("Rakesh","Panigrahi");
console.log(object);